And here comes some fun in the time when we all are locked inside our homes because of this COVID-19 pandemic ( 05/25/2020) . I am going to guide you on how you can build your own Tic_Tac_Toe Game using Python scripts in just few minutes and can enjoy playing with your loved once.
How to play Tic_Tac_Toe Game , Please watch the video here.
Tic_Tac_Toe Game : - Tic-tac-toe or noughts and crosses, or Xs and Os is a paper and pencil game for two players, X and O, who take turns marking the spaces in a 3×3 grid. The player who succeeds in placing three of their marks in a horizontal, vertical, or diagonal row is the winner. Here , instead of using paper and pencil we will use our computer/laptop to play this game.
The following example game is won by the first player, X:


Lets get started -
Below are the steps to create a Python Script to run Tic_Tac_Toe Game.
Step- 1 - Write a function that can take in the Player names using your keyboard.
def player_name(): Player_1 = input( "Player 1, Please Enter Your Name :").upper() Player_2 = input( "Player 2, Please Enter Your Name :").upper() return (Player_1 , Player_2)
Step- 2 - Write a function that can take in a player input and assign their marker as 'X' or 'O'.
def player_input(Player_1 , Player_2): marker='' while not (marker == 'X' or marker == 'O'): print("" +Player_1+ ": Do you want to be 'X' or 'O' ? ") marker = input().upper() if marker == 'X': return ('X','O') else: return ('O', 'X')
Step - 3- Write a function that can print out a board. Set up your board as a list, where each index 1-9 corresponds with a number on a number pad, so you get a 3 by 3 board representation.
def keypad_display(keypad_values): clear_output() # Remember, this only works in jupyter! print(' | | ') print(' ' + keypad_values[7] + ' | ' + keypad_values[8] + ' | ' + keypad_values[9]) print(' P(7)| P(8)|P(9)') print('------------------') print(' | | ') print(' ' + keypad_values[4] + ' | ' + keypad_values[5] + ' | ' + keypad_values[6]) print(' P(4)| P(5)|P(6)') print('------------------') print(' | | ') print(' ' + keypad_values[1] + ' | ' + keypad_values[2] + ' | ' + keypad_values[3]) print(' P(1)| P(2)|P(3)')
Step -4 - Write a function that uses the random module to randomly decide which player goes first.
def choose_first(): if random.randint(0,1) == 0: return Player_2 else: return Player_1
Step - 5- Write a function that takes in the board list object, a marker ('X' or 'O'), and a desired position (number 1-9) and assigns it to the board.
def place_marker(keypad_values,marker,position): keypad_values[position]=marker
Step -6 - Write a function that takes in a board and checks to see if someone has won.
def win_check(keypad_values,mark): return ((keypad_values[7] == keypad_values[8] == keypad_values[9] == mark) or (keypad_values[4] == keypad_values[5] == keypad_values[6] == mark) or (keypad_values[1] == keypad_values[2] == keypad_values[3] == mark) or (keypad_values[1] == keypad_values[5] == keypad_values[9] == mark) or (keypad_values[3] == keypad_values[5] == keypad_values[7] == mark) or (keypad_values[1] == keypad_values[4] == keypad_values[7] == mark) or (keypad_values[2] == keypad_values[5] == keypad_values[8] == mark) or (keypad_values[3] == keypad_values[6] == keypad_values[9] == mark))
Step -7 - Write a function that returns a boolean indicating whether a space on the board is freely available.
def space_check(keypad_values,position): return keypad_values[position]== ' '
Step -8 - Write a function that checks if the board is full and returns a boolean value.
def full_board_check(keypad_values): for i in range (1,10): if space_check(keypad_values,i): return False return True
Step - 9 - Write a function that asks for a player's next position (as a number 1-9) and then uses the function from step 7 to check if its a free position. If it is, then return the position for later use.
def player_choice(keypad_values, Player_name): position = 0 while position not in [1,2,3,4,5,6,7,8,9] or not space_check(keypad_values,position): print(""+Player_name+ " , Please Choose Your Next Position (1,9) ?") position = int(input()) return position
Step - 10 - Write a function that asks the player if they want to play again and returns a boolean True if they do want to play again.
def replay(): return input('Do you want to play again ? Enter yes or no ').lower().startswith('y')
Step - 11 - Here comes the REAL JOB !!! Make use of all the functions we have created inside a while loop and run the Game.
#Lets Play the Game !!! while True: keypad_values = [' ']*10 Player_1 , Player_2 = player_name() player1_marker,player2_marker = player_input( Player_1, Player_2) turn = choose_first() print(turn + " Will Go First ") play_game = input ( 'Are you ready to play the game ? Enter y or n : ') if play_game.lower()[0] == 'y': game_on = True else: game_on = False while game_on: if turn == Player_1: keypad_display(keypad_values) position = player_choice(keypad_values , Player_1) place_marker(keypad_values, player1_marker,position) if win_check(keypad_values,player1_marker): keypad_display(keypad_values) print(" Congratulations " +Player_1+"!!! You have Won the Game !!") game_on = False elif full_board_check(keypad_values): keypad_display(keypad_values) print('The Game is Draw!!') break else: turn = Player_2 else: keypad_display(keypad_values) position = player_choice(keypad_values, Player_2) place_marker(keypad_values, player2_marker,position) if win_check(keypad_values,player2_marker): keypad_display(keypad_values) print(" Congratulations " +Player_2+"!!! You have Won the Game !!") game_on = False elif full_board_check(keypad_values): keypad_display(keypad_values) print('The Game is Draw!!') break else: turn = Player_1 if not replay(): print(" Thank You for Playing , Hope You Enjoyed !!!") break
How to RUN the Script?
Well, here I am going to guide you how you can setup your environment and run this game.
Step -1 - Install Python in your computer.
Go to Python.org , Navigate to 'downloads' tab and Click on the latest version of Python available.
Step - 2 - Download the Tic_Tac_Toe_Game.zip file ,unzip it and get the Python Script from below.
Tic_Tac_Toe_Game
Step - 3 - Open the Command Prompt in your computer and Run the below command. ( Make sure you are running the script from correct directory)
python Tic_Tac_Toe_Game.py
Step - 4 - Enter your and your partner name , Choose what you want to pick up from either 'x' or 'o' and the game script will randomly choose which player will go first.

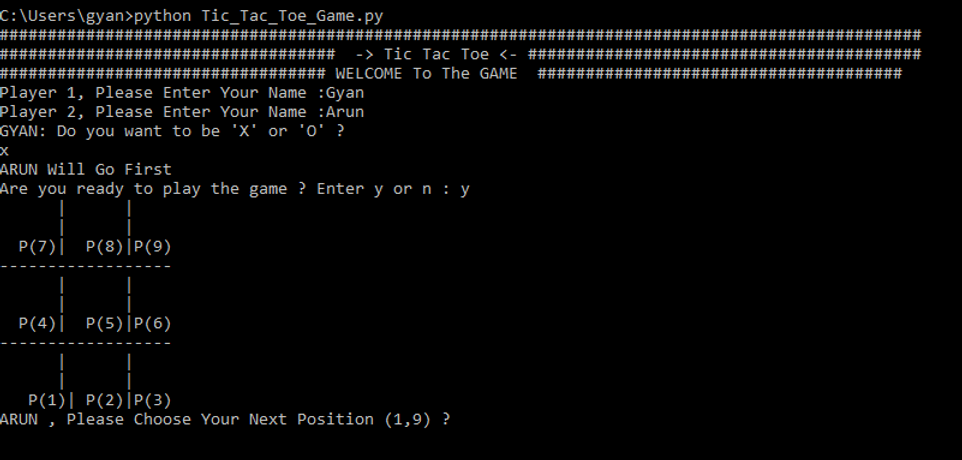
Step - 5 - Keep on playing till the game reaches to Winning position or a Draw.

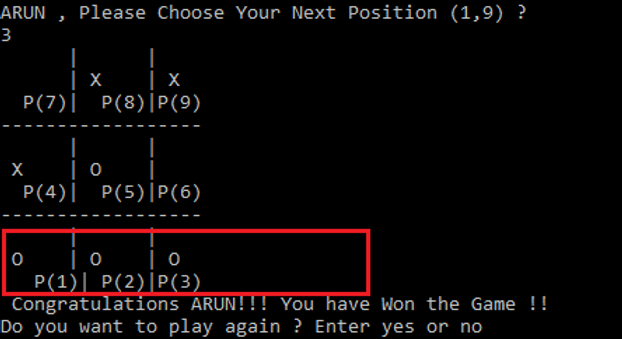
Step - 6 - Enter 'y' or 'n' if you want to play again or not.
------------------------------------------------------Thank You-----------------------------------------------------
If you face any issues while in understanding the script or running it , please put your query in the below comment section.
Happy Learning, Stay Safe !!!
0 Comments:
Post a Comment