CASINO !!! Yes , You imagined Rightly. Let's see how in couple of minutes you can develop your own Black Jack Game using Python Scripting.
Blackjack : Blackjack, formerly also Black Jack and Vingt-Un, is the American member of a global family of banking games known as "Twenty - One", whose relatives include Pontoon and Vingt-et -Un. It is a comparing card game between one or more players and a dealer, where each player in turn competes against the dealer. Players do not compete against each other. It is played with one or more decks of 52 cards, and is the most widely played casino banking game in the world. (Source : Wikipedia)
To know how to play Blackjack , please watch the video here.
Note: In this Project some of the Object Oriented Programming (OOP) concepts of Python along with basic Python Concepts are used. If you are new to Python , Please visit the Documentation page of www.python.org
Let's get started -
Here are the basic steps to play the game -
To play a hand of Blackjack the following steps must be followed-
Create a deck of 52 cards
Shuffle the deck
Ask the Player for their bet
Make sure that the Player's bet does not exceed their available chips
Deal two cards to the Dealer and two cards to the Player
Show only one of the Dealer's cards, the other remains hidden
Show both of the Player's cards
Ask the Player if they wish to Hit, and take another card
If the Player's hand doesn't Bust (go over 21), ask if they'd like to Hit again.
If a Player Stands, play the Dealer's hand. The dealer will always Hit until the Dealer's value meets or exceeds 17
Determine the winner and adjust the Player's chips accordingly
Ask the Player if they'd like to play again
Note: A standard deck of playing cards has four suits (Hearts, Diamonds, Spades and Clubs) and thirteen ranks (2 through 10, then the face cards Jack, Queen, King and Ace) for a total of 52 cards per deck. Jacks, Queens and Kings all have a rank of 10. Aces have a rank of either 11 or 1 as needed to reach 21 without busting.
Steps to Develop the GAME -
Step - 1 - Declare variables to store suits, ranks and values.suits = ('Hearts','Diamonds','Spades','Clubs') ranks = ('Two','Three','Four','Five','Six','Seven','Eight','Nine','Ten','Jack','Queen','King','Ace') values = {'Two':2,'Three':3,'Four':4,'Five':5,'Six':6,'Seven':7,'Eight':8,'Nine':9,'Ten':10,'Jack':10, 'Queen':10,'King':10,'Ace':11}
Step - 2 - Declare a Boolean value to be used to control while loops.playing = True
Step - 3 - Create a 'card' class which hold two attributes suit and rank. Also , In addition to the Card's __init__ method, consider adding a __str__ method ( Special Methods) that, when asked to print a Card, returns a string in the form "Two of Hearts" ( These are special methods for Python class)class Card(): def __init__(self,suit,rank): self.suit = suit self.rank = rank def __str__(self): return self.rank + '_of_' + self.suit
Step -4 - Create a Deck Class, Here we might store 52 card objects in a list that can later be shuffled. First, though, we need to instantiate all 52 unique card objects and add them to our list. So long as the Card class definition appears in our code, we can build Card objects inside our Deck __init__ method. In addition to an __init__ method we'll want to add methods to shuffle our deck, and to deal out cards during game play.
class Deck(): def __init__(self): self.deck = [] for suit in suits: for rank in ranks: self.deck.append(Card(suit,rank)) def __str__(self): deck_comp = '' for card in self.deck: deck_comp +='\n'+card.__str__() return 'The deck has:' + deck_comp def shuffle(self): random.shuffle(self.deck) def deal(self): single_card = self.deck.pop() return single_card
Step - 5 - Create a Hand Class, In addition to holding Card objects dealt from the Deck, the Hand class may be used to calculate the value of those cards using the values dictionary defined above. It may also need to adjust for the value of Aces when appropriate.
class Hand(): def __init__(self): self.cards = [] self.value = 0 self.aces = 0 def add_card(self,card): self.cards.append(card) self.value += values[card.rank] if card.rank == 'Ace': self.aces +=1 def adjust_for_ace(self): if self.value >21 and self.aces: self.value -=10 // Adding one because according to 'Ace' value in dictionary , 11 will be added first. self.aces -=1
Step - 6 - Create a Chips Class, In addition to decks of cards and hands, we need to keep track of a Player's starting chips, bets, and ongoing winnings. This could be done using global variables, but in the spirit of object oriented programming, let's make a Chips class instead!
class Chips(): def __init__(self,total): self.total = total self.bet = 0 def win_bet(self): self.total +=self.bet def lose_bet(self): self.total -= self.bet
Step -7 - Write a function for taking bets , Since we're asking the user for an integer value, this would be a good place to use try/except( Special blocks to handle the Code ). Remember to check that a Player's bet can be covered by their available chips.
def take_bet(chips): while True: try: chips.bet =int(input("How many chips would you like to bet ?")) except valueError: print('Sorry!! A bet must be an integer') else: if chips.bet > chips.total: print("Sorry, Your bet can't exceed", chips.total) else: break
Step - 8 - Write a function for taking hits, Either player can take hits until they bust. This function will be called during game play anytime a Player requests a hit, or a Dealer's hand is less than 17. It should take in Deck and Hand objects as arguments, and deal one card off the deck and add it to the Hand. You may want it to check for aces in the event that a player's hand exceeds 21. def hit(deck,hand): hand.add_card(deck.deal()) hand.adjust_for_ace()
Step - 9 - Write a function prompting the Player to Hit or Stand, This function should accept the deck and the player's hand as arguments, and assign playing as a global variable.
If the Player Hits, employ the hit() function above. If the Player Stands, set the playing variable to False - this will control the behavior of a while loop later on in our code.def hit_or_stand(deck,hand): global playing while True: x = input("Would you like to Hit or Stand? Enter 'H' or 'S' ") if x[0].lower() == 'h': hit(deck,hand) elif x[0].lower() == 's': print('player stands, dealer is playing') playing = False else: print('Sorry, please try again!!') continue break
Step -10 - Write functions to display cards, When the game starts, and after each time Player takes a card, the dealer's first card is hidden and all of Player's cards are visible. At the end of the hand all cards are shown, and you may want to show each hand's total value. Write a function for each of these scenarios.def show_some(player,dealer): print("\n Dealer's hand:") print('<Card Hidden>') print('',dealer.cards[1]) print("\nPlayer's Hand:",*player.cards , sep='\n') def show_all(player,dealer): print("\nDealer's Hnad:",*dealer.cards , sep='\n') print("Dealer's Hand =", dealer.value) print("\nPlayer's Hand:",*player.cards , sep="\n") print("Player's Hand =",player.value)
Step - 11 -Write functions to handle end of game scenarios, Remember to pass player's hand, dealer's hand and chips as needed.
def player_busts(player,dealer,chips): print("Player Busts!!") chips.lose_bet() def player_wins(player,dealer,chips): print("Player Wins!!") chips.win_bet() def dealer_busts(player,dealer,chips): print("Dealer Busts!!") chips.win_bet() def dealer_wins(player,dealer,chips): print("Dealer Wins!!") chips.lose_bet() def push(player,dealer): print("Dealer and Player tie!!It's a PUSH")
Step -12 - Final Game !!while True: print("Welcome to BlackJack!!!") deck = Deck() deck.shuffle() player_hand = Hand() player_hand.add_card(deck.deal()) player_hand.add_card(deck.deal()) dealer_hand = Hand() dealer_hand.add_card(deck.deal()) dealer_hand.add_card(deck.deal()) player_chips = Chips(100) take_bet(player_chips) show_some(player_hand,dealer_hand) while playing: hit_or_stand(deck,player_hand) show_some(player_hand,dealer_hand) if player_hand.value > 21: player_busts(player_hand,dealer_hand,player_chips) break if player_hand.value<= 21: while dealer_hand.value < 17: hit(deck,dealer_hand) show_all(player_hand,dealer_hand) if dealer_hand.value > 21: dealer_busts(player_hand,dealer_hand,player_chips) elif dealer_hand.value > player_hand.value: dealer_wins(player_hand,dealer_hand,player_chips) elif dealer_hand.value < player_hand.value: player_wins(player_hand,dealer_hand,player_chips) else: push(player_hand,dealer_hand) print("Player's Winnings Stands At",player_chips.total) new_game = input("Would you like to play again? Say Yes or No ") if new_game[0].lower() == 'y': playing = True continue else: print("Thanks for Playing!!") break
How to RUN the Script?
Well, here I am going to guide you on how you can setup your environment and run this game in your windows system.
Step -1 - Install Python in your computer.
Go to Python.org , Navigate to 'downloads' tab and Click on the latest version of Python available.
Step - 2 - Download the BlackJack.zip file ,unzip it and get the Python Script ready for the Game from below.
BlackJack
Step - 3 - Open the Command Prompt in your computer and Run the below command. ( Make sure you are running the script from correct directory)
python BlackJack.py
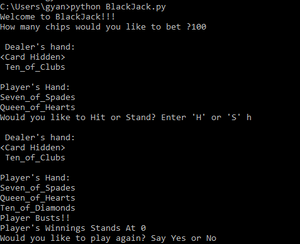
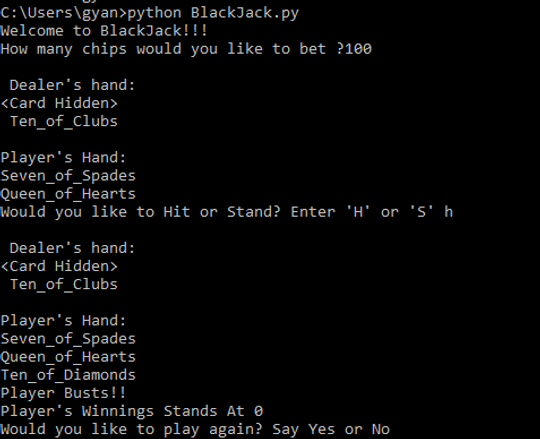
Step - 4 - Enjoy the Game !!!
----------------------------------------------------Thank You--------------------------------------------------------
If you face any issues while in understanding the script or running it , please put your query in the below comment section.
Happy Learning, Stay Safe !!!
0 Comments:
Post a Comment